C Program To Print Number Is Even or Odd Without Using "if-else" Statement Using "Switch-Case"
Program to Print number is even or odd without using if-else statement using switch case:
#include<stdio.h>
int main()
{
int no;
printf("enter the no\n");
scanf("%d",&no);
#include<stdio.h>
int main()
{
int no;
printf("enter the no\n");
scanf("%d",&no);
C Program To Print Maximum Number Between Two Numbers Using Switch Case
Ex: write a C program to find maximum number between two numbers without using if-else using switch case. How to write a C program to find maximum number between two numbers without using if-else using switch case.
c program to print maximum out of two numbers without using if-else statement using Switch Case.
Input from user:
Enter value of a: 5
Enter value of b: 15
Expected Output:
Maximum is b=15.
In below code we will print max out of two numbers without using if-else statement's. Here we will use switch-case to find the max number of given two numbers.
Step by Step Logic of the program:
1. First we will accept two numbers from user which say a & b.
2. After that we will store the output of a/b in the switch. Switch (a/b)
3. If output in zero then case 0 will work means max is b.
4. Otherwise in default- a is max.
#include<stdio.h>
int main()
{
int a,b;
printf("enter value of a\n");
scanf("%d",&a);
printf("enter value of b\n");
scanf("%d",&b);
switch(a/b)
{
case 0:
printf("max is b=%d",b);
break;
default:
printf("max is a=%d",a);
}
return 0;
}
Above Program show's the following output:
c program to print maximum out of two numbers without using if-else statement using Switch Case.
Input from user:
Enter value of a: 5
Enter value of b: 15
Expected Output:
Maximum is b=15.
In below code we will print max out of two numbers without using if-else statement's. Here we will use switch-case to find the max number of given two numbers.
Step by Step Logic of the program:
1. First we will accept two numbers from user which say a & b.
2. After that we will store the output of a/b in the switch. Switch (a/b)
3. If output in zero then case 0 will work means max is b.
4. Otherwise in default- a is max.
C Program To Print Maximum Number Between Two Numbers Using Switch Case:
#include<stdio.h>
int main()
{
int a,b;
printf("enter value of a\n");
scanf("%d",&a);
printf("enter value of b\n");
scanf("%d",&b);
switch(a/b)
{
case 0:
printf("max is b=%d",b);
break;
default:
printf("max is a=%d",a);
}
return 0;
}
Above Program show's the following output:
C Program To Reverse the String without using "strrev()" function
Ex: Write a C Program To Reverse the String without using "strrev()" function. How to write a C Program To Reverse the String without using "strrev()" function. C Program To Reverse the String without using "strrev()" function.
Program:
#include<stdio.h>
#include<string.h>
void main()
{
char a[100],b[100];
int i,j,len;
printf(" \n Please Enter Any String : ");
gets(a);
j=0;
#include<stdio.h>
#include<string.h>
void main()
{
char a[100],b[100];
int i,j,len;
printf(" \n Please Enter Any String : ");
gets(a);
j=0;
/*Calculate length of string using strlen() function and store result in variable len*/
len=strlen(a);
len=strlen(a);
//Run for loop from len-1 to 0.
for(i=len-1;i>=0;i--)
{
C Program to Reverse the Given String Using String Function
Ex: Write a C program to reverse the given string using String function. How to write a C program to reverse the given string using String function. C program to reverse the given string using String function.
Program:
#include <stdio.h>
#include <string.h>
void main()
{
char s[100];
//Accept string from user using gets() function
printf("\n Please Enter Any String : ");
gets(s);
//Reverse the given string using strrev() function
strrev(s);
//Print reversed string on the output screen
printf("\n string After Reversing : %s\n", s);
}
Above Program show's the following output:
#include <stdio.h>
#include <string.h>
void main()
{
char s[100];
//Accept string from user using gets() function
printf("\n Please Enter Any String : ");
gets(s);
//Reverse the given string using strrev() function
strrev(s);
//Print reversed string on the output screen
printf("\n string After Reversing : %s\n", s);
}
Above Program show's the following output:
C Program To Print Sum Of Two Numbers Without Using Arithmetic '+' Operator
Ex: Write a C Program To Print Sum Of Two Numbers Without Using Arithmetic '+' Operator. How to write a C Program To Print Sum Of Two Numbers Without Using Arithmetic '+' Operator. C Program To Print Sum Of Two Numbers Without Using Arithmetic '+' Operator.
Input from user:
Enter number 1: 10
Enter number 2: 5
Expected output:
Sum = 15
Program:
#include<stdio.h>
void main()
{
int no1,no2,no3;
printf("enter no1\n");
scanf("%d",&no1);
printf("enter no2\n");
scanf("%d",&no2);
C Program to Print Alternate Prime Numbers from 1 to n
Ex: Write a C program to print alternate prime numbers from 1 to n. How to write a C program to print alternate prime numbers from 1 to n. C program to print alternate prime numbers from 1 to n.
Input from user:
Enter the number: 100
Expected output:
2 5 11 17 23 31 41 47 59 67 73 83 97
- What is prime number?
Prime number means a number that is divisible only by 1 and itself.
Step by step logic of the given program:
1. Accept input from user declare variable say no.
2. Declare e=2.
3. Run one outer for loop from 1 to no:
for(i=1;i<=no;i++)
4. Run one inner for loop from 1 to i and inside that check condition if i%j == 0 then increment c by 1:
for(j=1;j<=i;j++)
{
if(i%j==0)
{
c++;
}
}
5. After that outside the inner for loop check if c==2 (it means if given number is prime) then, inside that if-statement check one more condition if e%2==0 means if e is even number then print value of i (it prints prime numbers only if e is completely divisible by 2, in short it prints alternate prime numbers):
if(c==2)
{
if(e%2==0)
{
printf("%d ",i);
}
6. After that, Outside the block of inner if statement increment value of e by 1.
7. Last make c=0.
Program to Print Alternate Prime Numbers:
#include<stdio.h>
void main()
{
int no,i,j,c=0,e;
printf("Enter the number:\n");
scanf("%d",&no);
e=2;
printf("Alternate Prime Number's Are:\n");
for(i=1;i<=no;i++)
{
for(j=1;j<=i;j++)
{
if(i%j==0)
{
c++;
}
}
if(c==2)
{
if(e%2==0)
{
printf("%d ",i);
}
e=e+1;
}
c=0;
}
}
Above Program show's the following output:
C program to print following numbers series 7.5 9.5 15.5 27.5 47.5
Ex: Write a C program to print following numbers series 7.5 9.5 15.5 27.5 47.5...How to write a C program to print following numbers series 7.5 9.5 15.5 27.5 47.5.....C program to print following numbers series 7.5 9.5 15.5 27.5 47.5.
Input from user:
Enter a number: 5
Expected output:
7.5 9.5 15.5 27.5 47.5
Program:
#include<stdio.h>
void main()
{
int i,no;
float z=7.5;
#include<stdio.h>
void main()
{
int i,no;
float z=7.5;
printf("Enter a number:\n");
scanf("%d",&no);
C program to devide the intiger number without using division "/" sign
Ex: Write a C program to devide the intiger number without using division "/" sign. How to write a C program to devide the intiger number without using division "/" sign. C program to devide the intiger number without using division "/" sign.
Input from user:
Enter number1: 25
Enter number2: 5
Expected output:
Division is 5
Program:
#include<stdio.h>
void main()
{
int no1,no2,c=1;
#include<stdio.h>
void main()
{
int no1,no2,c=1;
printf("enter the no1\n");
scanf("%d",&no1);
printf("enter the 2nd no\n");
scanf("%d",&no2);
C Program to print your name 10 times without using while loop, for loop or goto statement
Ex: Write a C Program to print your name 10 times without using loops or goto statement using recursion function. How to write a C Program to print your name 10 times without using loops or goto statement using recursion function. C Program to print your name 10 times without using loops or goto statement using recursion function.
In below program we have used recursion function to print name 10 times.
What is recursion function?
Recursion function means function calling it self ...In simple words recursion() means call a function inside the same function then it is called as recursive call of the function.
C Program to Delete the Element from Array
Ex: Write a C program to delete the element from array. How to write a C program to delete the element from array. C program to delete the element from array.
Input from user:
Enter how many elements you want: 7
Enter the elements:
1
2
3
4
2
5
6
Entered elements are:
1 2 3 4 2 5 6
Enter which element you want to delete: 2
Enter the position: 5
Expected ouput:
After deleting, array element's are:
1 2 3 4 5 6
Program:
Above Program shows the following output:
#include<stdio.h>
void main()
{
int a[100],i,no,key,temp,pos;
//Accept limit from user..
printf("Enter how many elements you want:\n");
scanf("%d",&key);
//Accepting elements
printf("\nEnter the elements:\n");
for(i=0;i<key;i++)
scanf("%d",&a[i]);
/*Print entered elements*/
printf("Entered elements are:\n");
for(i=0;i<key;i++)
printf("%d ",a[i]);
/*Accept element which want to delet*/
printf("\nEnter which element you want to delet:\n");
scanf("%d",&no);
/*Accept position of element*/
printf("Enter the position:\n");
scanf("%d",&pos);
/*logic to delet element*/
for(i=pos-1;i<=key;i++)
{
a[i]=a[i+1];
}
/*print elements after deleting*/
printf("After deleting, array elements are:\n");
for(i=0;i<key-1;i++)
printf("%d ",a[i]);
}
Above Program shows the following output:
C Program to Print Swastika Star Pattern
Ex: Write a C Program to print swastika star pattern. How to write a C Program to print swastika star pattern. C Program to print swastika star pattern.
Input from user:
Enter the number:
5
Expected output:
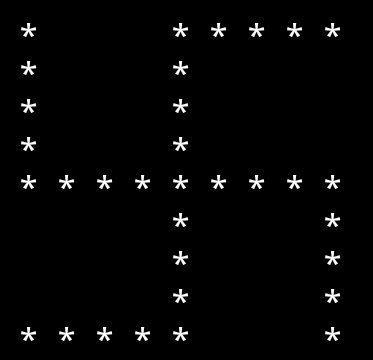
Step by Step logic of the given program:
1. Accept input number from user declare variable say no.
2. Run outer for loop from 1 to (no*2-1):
for(i=1;i<=no*2-1;i++)
3. Run inner for loop from 1 to (no*2-1):
for(j=1;j<=no*2-1;j++)
4. Use if statement for add conditions to print stars:
if(i==no||j==no||i==1&&j>no||i<no&&j==1||i>no&&j==no*2-1||i==no*2-1&&j<no)
{
printf(" *");
}
5. Otherwise print spaces. For next row print new line(\n).
C Program to Print Swastika Star Pattern:
#include<stdio.h>
void main()
{
/* Declare variables*/
int i,j,no;
printf("Enter the number:\n");
scanf("%d",&no);
/* Run loops*/
for(i=1;i<=no*2-1;i++)
{
for(j=1;j<=no*2-1;j++)
{
/* Conditions for print stars*/
if(i==no||j==no||i==1&&j>no||i<no&&j==1||i>no&&j==no*2-1||i==no*2-1&&j<no)
{
printf(" *");
}
else
{
printf(" ");
}
}
printf("\n");
}
}
Above Program shows the following output:
C Program To Print Pyramid Star Pattern
Ex: Write a C program to print pyramid star pattern. How to write a C program to print pyramid star pattern. C program to print pyramid star pattern.
Input from user:
Enter the number:
7
Expected output:
*
**
***
****
*****
******
*******
Step by step logic of the given program:
1. Accept input (number) from user which shows size of the pyramid, declare variable say no.
2. Run one outer for loop from 1 to no:
for(i=1;i<=no;i++)
3. To print spaces run another for loop from i to no-1:
for(j=i;j<no;j++)
{
printf(" ");
}
4. To print star run another one inner for loop from 1 to i:
for(k=1;k<=i;k++)
{
printf(" *");
}
5. For new row, move to next line using \n.
Program:
#include<stdio.h>
void main()
{
int i,j,k,no;
printf("Enter the number:\n");
scanf("%d",&no);
for(i=1;i<=no;i++)
{
/*Print spaces*/
for(j=i;j<no;j++)
{
printf(" ");
}
/*print star*/
for(k=1;k<=i;k++)
{
printf(" *");
}
/*Move to the next line*/
printf("\n");
}
}
C Program to print hollow diamond star pattern
Ex: Write a C Program to print hollow diamond star pattern. How to write a C Program to print hollow diamond star pattern. C Program to print hollow diamond star pattern.
We will print this pattern in two parts, first we print upper half of the pattern then we print below, half part of pattern.
Step by step logic of the given program:
1. Accept input (number) from user declare variable say no.
2. print upper half of the pattern:
**********
**** ****
*** ***
** **
* *
a). Run one outer for loop from 1 to no.
b). After that run inner loop from i to no to print inverted right triangle pattern.
c). Run another one inner loop from 1 to i*2-2 to print spaces.
d). Last run one more inner loop from i to no to print another inverted right triangle and to complete right side of upper half pattern.
Inside the outer loop, for new row, print new line (\n).
3. Print lower half of the pattern:
* *
** **
*** ***
**** ****
**********
a). Run one outer for loop from 1 to no.
b). Inside the outer loop run inner loop from 1 to i to print right triangle pattern.
c). Run another one inner loop from 1 to (no*2-i*2) to print spaces.
d). Last run one more inner loop from 1 to i to print another right triangle and to complete right side of lower half pattern.
Inside the outer loop, for new row, move to next line using \n.
Program:
#include<stdio.h>
void main()
{
int no;
int i,j;
printf("Enter the number:\n");
scanf("%d",&no);
/*loop to print upper half pattern*/
for(i=1;i<=no;i++)
{
for(j=i;j<=no;j++)
{ printf("*");
}
for(j=1;j<=(i*2-2);j++)
{
printf(" ");
}
for(j=i;j<=no;j++)
{
printf("*");
}
printf("\n");
}
/*loop to print lower half of the pattern*/
for(i=1;i<=no;i++)
{
for(j=1;j<=i;j++)
{
printf("*");
}
for(j=1;j<=(no*2-i*2);j++)
{ printf(" ");
}
for(j=1;j<=i;j++)
{
printf("*");
}
printf("\n");
}
}
Above Program show's the following output:
Subscribe to:
Posts (Atom)